Logging
YASL handles logging through the Simple Logging Facade for Java (SLF4J; see http://www.slf4j.org). The current release of YASL is using version 1.4.3 of the SLF4J library. SLF4J can be configured to work with many different logging packages by placing the appropriate adapter or implementation jar on the application's classpath.
Using SLF4J
Using SLF4J is easy. To obtain a logger for a class, use the following:
import org.slf4j.Logger; import org.slf4j.LoggerFactory; ... private final Logger logger = LoggerFactory.getLogger(YourClassName.class);The Logger interface is extensive. A sample of the API is listed below:
logger.info("entering performAction method"); logger.debug("a debug message"); logger.warn("a warning message"); logger.error("an error message"); try { throw new Exception("dummy exception"); } catch(Exception ex) { logger.error("error message with stack trace", ex); } logger.info("formatted {} message.", "info"); logger.info("formatted {} message with {} arguments.", "info", new Integer(2));Where the log messages appear (file, console, etc.) and the logging level (debug, info, warn, or error) depend on the particular implementation or adaptor being used.
Jars Required
SLF4J requires at least two jars to be on your application's classpath. Combinations for a few implementations are listed below:
For the SLF4J No-Op Logger
- slf4j-api-1.4.3.jar
- slf4j-nop-1.4.3.jar
For the SLF4J Simple Logger
- slf4j-api-1.4.3.jar
- slf4j-simple-1.4.3.jar
For the YASL SLF4J Logger
- slf4j-api-1.4.3.jar
- yasl-slf4j-1.1.jar
YASL's Logging Component
If an application uses the YASL SLF4J Logger, the application will be able to use the YASL Log Manager. This component provides access to all the SLF4J loggers in the application. Users can set the logging level (DEBUG, INFO, WARN, ERROR) and logging location (Console, Log File, Log Manager) for each logger. If the logging location is set to Log Manager, the messages from the logger will appear in the Log Manager component's text window. The Log Manager is a non-modal dialog so users can interact with the rest of the application while the Log Manager is open.
YASLLoggingComponentAction
(from org.yasl.logging.action
) is a ready-to-use action class for invoking the Log Manager. The following code examples for the Log Manager config are from the YASL Test Application.
From yaslTestAppConfig.xml:
<action class="org.yasl.logging.action.YASLLoggingComponentAction" key="logging_component"> <argRBString bundle="org.yasl.testapp.resources.yaslTestAppGuiStrings" key="menu.item.loggingcomp"/> <argPrimitive type="boolean" value="true"/> <argKeyedObject keyType="singletons" key="yasl_application" class="org.yasl.arch.application.YASLSwingApplication"/> </action>From yaslTestAppMenuConfig.xml:
<menu key="menu_component"> <argRBString bundle="org.yasl.testapp.resources.yaslTestAppGuiStrings" key="menu.test3"/> </menu> ... <menuItem attachTo="menu_component"> <argKeyedObject keyType="actions" key="logging_component" class="javax.swing.Action"/> </menuItem>
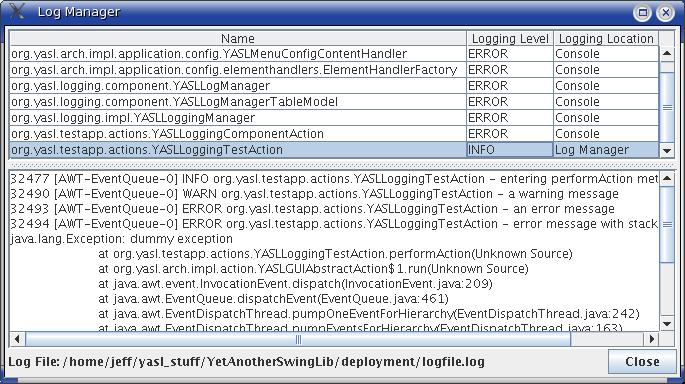
Image 1: YASL Log Manager
Configuring the YASL Implementation
The YASL SLF4J implementation has numerous configurable properties. These properties can be configured from the command line or a properties file. Below is a list of the configurable properties:
- logging.level
Sets the initial level for loggers.
Values: error, warn, info, debug
Default value: error - use.single.logger
Controls if the logger factory uses a single logger instance for the application or a separate logger instance for each class requesting a logger.
Values: true, false
Default value: false - logging.target
Defines the place where log messages will be recorded.
Values: logmanager, file, console
Default value: console - use.logging.file
If set to false, a logfile using the logging.file name will not be created. Messages will only be logged to the log manager or console.
Values: true, false
Default value: true - logging.file
Name of file to which messages will be logged when logging.target is set to file. '.log' will be appended to the file name when it is created.
Values: valid file names
Default value: logfile - logging.file.append
Controls if log messages should be appended to the existing log file. If set to false and a log file already exists, a new log file with an integer appended to its name will be used.
Values: true, false
Default value: true - logging.file.append.date
If set to true, appends the date to the file name, using MM-DD-YYYY.
Values: true, false
Default value: false
To configure a property from the command line, set the property as a system property at JVM start-up. For example, to set the initial logging level for all loggers to info
, use the following: java -Dlogging.level=info
.
To configure properties from a property file, create a file called yaslslf4jlogging.properties and place it in your application's classpath. This file should not be inside a package within your application.